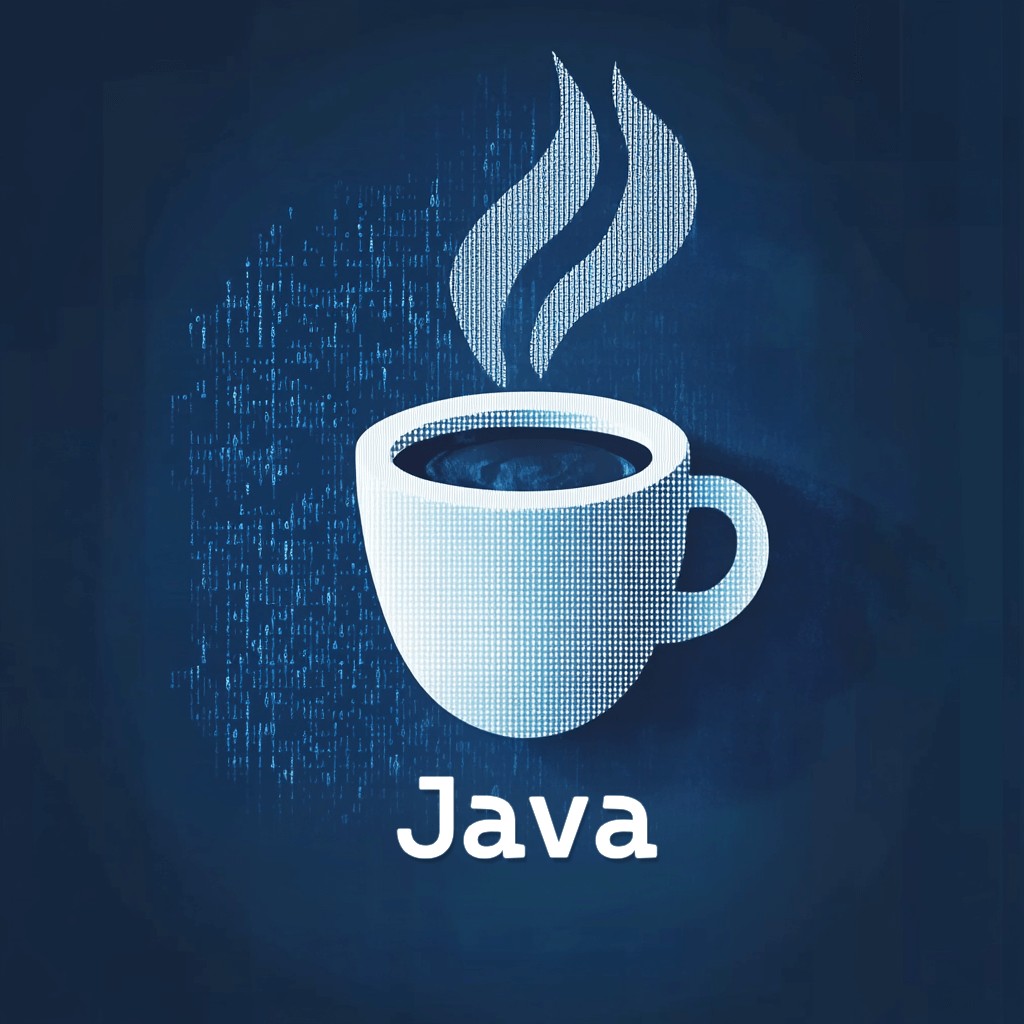
Understanding the structure of a Java program is essential for writing clean, organized, and functional code. Let’s break down each component of a Java program and see how it all fits together.
Package
package org.example;
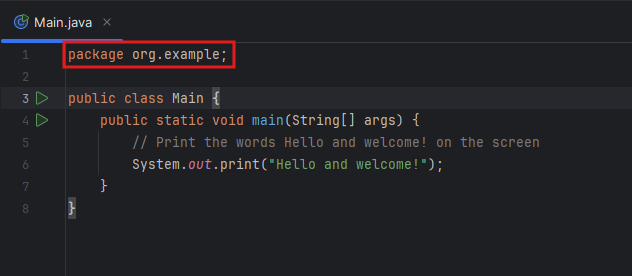
This statement tells the compiler that the Java file we’ve written belongs to the org.example
package.
What is a Package?
A package is a way to group related classes and interfaces together. Think of it as a folder that organizes your files.
Benefits of Using Packages:
- Avoid Naming Conflicts: Multiple files with the same name can exist in a project as long as they are in different packages.
- Code Organization: Packages make it easier to find, use, and manage classes in a large project.
This is similar to how we organize files on our computer into separate folders to avoid duplication issues.
The Main Class
Next, let’s look at the Main class:
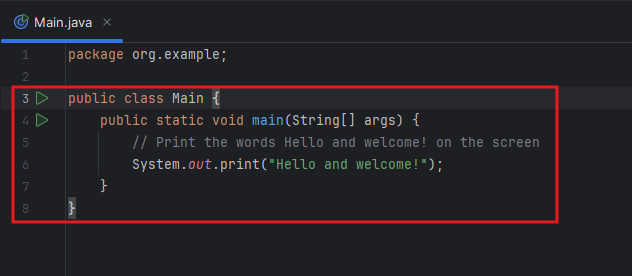
The Main class starts on line 3 with an opening curly brace {
and ends on line 8 with a closing curly brace }
.
Curly braces are used in Java to indicate the beginning and end of code blocks.
The main()
Method
Inside the Main class, we have the main()
method:
public static void main(String[] args) {
// Print the words Hello and welcome! on the screen
System.out.print("Hello and welcome!");
}
What is the main()
Method?
The main()
method is the entry point of all Java applications. This is the first method that the Java Virtual Machine (JVM) calls when executing a program.
Key Details About main()
:
- It contains
String[] args
, meaning it can take an array of strings as input. - It acts as a bridge between the user and the program.
Analyzing Our Example Code:
1. Comments in main()
:
The line:
// Print the words Hello and welcome! on the screen
is a comment. Comments are ignored by the compiler and are used to make the code more understandable for developers.
2. Printing Output:
The line:
System.out.print("Hello and welcome!");
Important Note: All Java statements, such as System.out.print, must end with a semicolon (;).
displays the text “Hello and welcome!” on the output window.
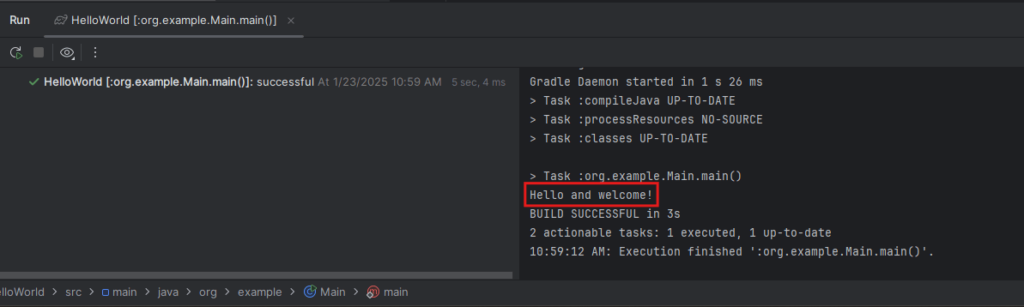
Comments in Java
Comments are an essential part of programming. They improve code readability and serve as notes for other programmers. Comments are not compiled into bytecode and do not affect the program’s functionality.
Examples of Comments:
Single-line Comments:
Single-line comments begin with //
. Examples:
// This is a single-line comment
// Display a welcome message
// Initialize variables
Multi-line Comments:
Multi-line comments begin with /*
and end with */
. Examples:
/* This is a
multi-line comment */
Another multi-line comment example:
/*
Define variables and methods
to implement functionality
*/
Inline Comments:
Comments can also appear on the same line as code:
System.out.print("Hello and welcome!"); // Print the words Hello and welcome! on the screen
In this post, we explored the structure of a Java program, from packages and classes to the main()
method and comments. These foundational elements are essential for understanding Java programming and writing efficient code.