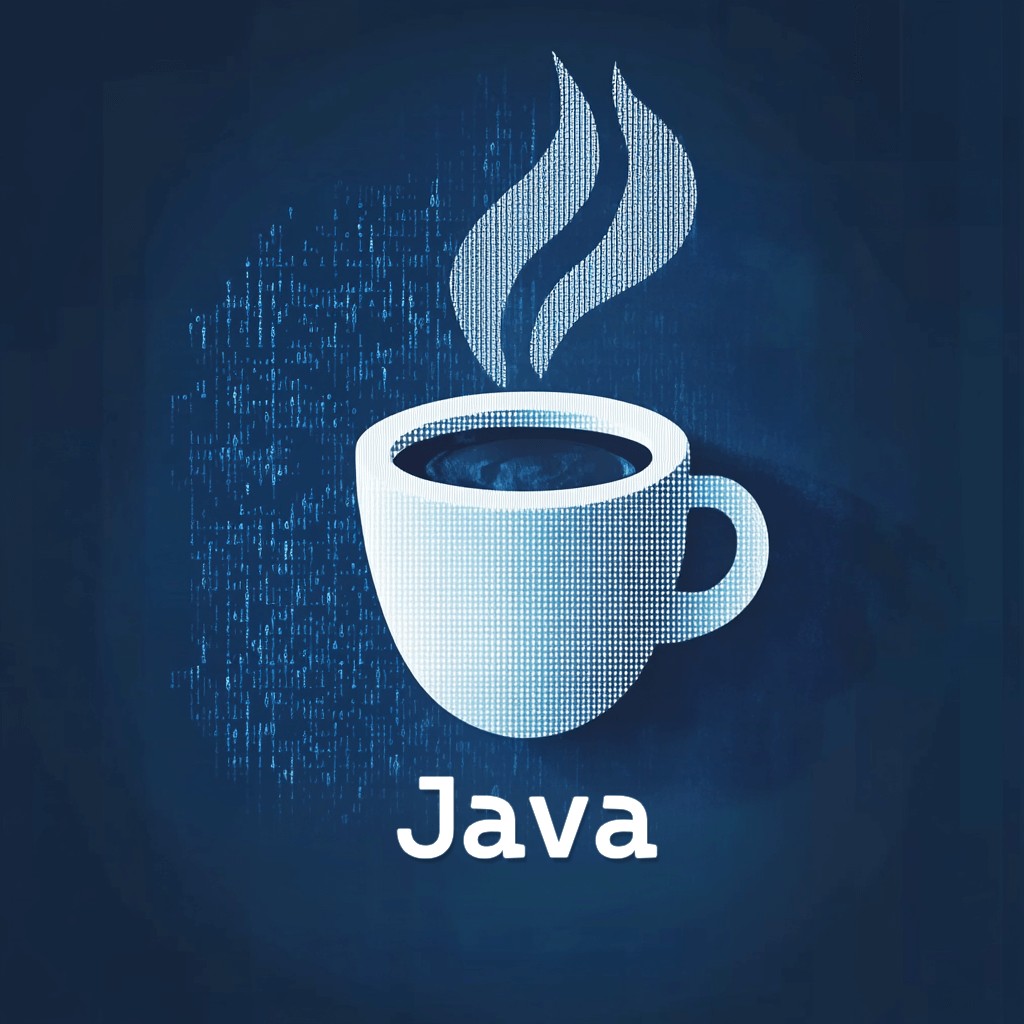
In this post, we’ll explore two fundamental building blocks of Java programming: variables and operators. By the end, you’ll have a clear understanding of what variables are, how to work with them, and the basic operations you can perform.
What Are Variables?
At its core, a variable is like a labeled container in your program that holds data you want to use and manipulate. Think of it as a storage box with a name on it, where you can keep different types of items (data).
Example: Suppose you want to store the number of points a player has earned in a game. You can create a variable named points
like this:
int points;
This line does two things:
- Data Type (
int
): Specifies thatpoints
will hold integer values (whole numbers). - Variable Name (
points
): The identifier you’ll use to reference this data in your code.
Once declared, your program reserves memory to store the value of points
. You can then assign a value to it and update it as needed:
points = 10; // Assigns the value 10 to points
points += 5; // Increases points by 5, making it 15
Using variables helps your program manage data efficiently, making your code more flexible and easier to maintain.
Primitive Data Types in Java
Java offers 8 primitive data types, which are the building blocks for data manipulation in your programs. These types are divided into two main categories: integer types and floating-point types, along with char
and boolean
.
Integer Types
1. byte
- Description: Stores small integer values.
- Range: -128 to 127
- Size: 1 byte
2. short
- Description: Stores slightly larger integer values.
- Range: -32,768 to 32,767
- Size: 2 bytes
3. int
- Description: Commonly used for integer values.
- Range: -2,147,483,648 to 2,147,483,647
- Size: 4 bytes
4. long
- Description: Used for very large integer values.
- Range: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
- Size: 8 bytes
- Usage Note: Append an uppercase
L
to denote a long literal.
long distance = 100000L;
Floating-Point Types
5. float
- Description: Represents single-precision 32-bit IEEE 754 floating-point numbers.
- Range: Approximately -3.40282347 × 10³⁸ to 3.40282347 × 10³⁸
- Precision: Up to 7 decimal digits
- Usage Note: Append an uppercase
F
to denote a float literal.
float temperature = 36.6F;
6. double
- Description: Represents double-precision 64-bit IEEE 754 floating-point numbers.
- Range: Approximately -1.7976931348623157 × 10³⁰⁸ to 1.7976931348623157 × 10³⁰⁸
- Precision: Up to 15 decimal digits
- Usage Note: By default, floating-point literals are considered
double
. To specify afloat
, use theF
suffix.
double pi = 3.141592653589793;
Other Primitive Types
7. char
- Description: Stores single Unicode characters like
'A'
,'@'
, or'3'
. - Size: 2 bytes
char grade = 'A';
8. boolean
- Description: Represents one of two values:
true
orfalse
. - Usage: Commonly used for conditional statements and logic control.
boolean isJavaFun = true;
Naming a Variable
Choosing the right name for your variables is essential for writing clear and maintainable code. Java has specific rules and conventions to help you name variables effectively.
Allowed Characters
- Letters: Both uppercase (
A-Z
) and lowercase (a-z
). - Digits: Numbers (
0-9
), but cannot be the first character. - Underscore (
_
) and Dollar Sign ($
): These symbols are permitted but are generally avoided unless necessary.
Valid Variable Names:
__number
$number
number
number2
Invalid Variable Name:
2number
(Cannot start with a digit)
Naming Conventions
1. Start with a Letter: While Java allows starting with _
or $
, it’s standard practice to begin with a letter to enhance readability.
2. Meaningful and Concise: Choose names that clearly describe the variable’s purpose.
Good Examples:
username
totalScore
Poor Examples:
a
(Too vague)
data123
(Not descriptive)
Restrictions
- Reserved Words: Java has reserved keywords (e.g.,
if
,else
,while
,class
,public
) that cannot be used as variable names.
Invalid Examples:
int if; // Error: Uses reserved word 'if'
boolean while; // Error: Uses reserved word 'while'
Case Sensitivity
Java is case-sensitive, meaning that variables with names differing only in letter case are considered distinct.
Examples:
- ifThisIsOn
- ifthisison
In this case, ifThisIsOn
and ifthisison
are two separate variables.
Initializing a Variable
After declaring a variable, it’s important to assign it an initial value. This process is called initializing the variable. You can initialize a variable either at the time of declaration or separately in a distinct statement.
1. Initializing at the Point of Declaration
Assign a value to a variable immediately when you declare it. Here are some examples:
int employeeCount = 300;
String username = "James";
byte experienceYears = 10;
short studentCount = 35;
long population = 11231314110863L;
float ratePerHour = 65.5F;
double totalHours = 5236.5;
char performanceGrade = 'A';
boolean isPromoted = true;
Notes:
long
Literals: Append an uppercaseL
(e.g.,11231314110863L
).float
Literals: Append an uppercaseF
(e.g.,65.5F
).char
Values: Enclosed in single quotes (e.g.,'A'
).String
Type: Initialized with double quotes (e.g.,"James"
).
2. Declaring Multiple Variables of the Same Type
You can declare multiple variables of the same type in a single statement, separating each with a comma:
boolean isPromoted = true, isUsed = false;
Explanation:
isPromoted
is initialized totrue
.isUsed
is initialized tofalse
.
3. Initializing Variables in Separate Statements
Alternatively, declare a variable first and then assign it a value separately:
int employeeCount;
employeeCount = 300;
Steps:
- Declaration:
int employeeCount;
– DeclaresemployeeCount
as an integer. - Initialization:
employeeCount = 300;
– Assigns the value300
toemployeeCount
.
The Assignment Operator (=
)
In programming, the =
symbol is known as the assignment operator. It’s used to assign values to variables.
Basic Example
Consider two integer variables, x
and y
:
int x = 5; // Declares x and initializes it to 5
int y = 10; // Declares y and initializes it to 10
Using the Assignment Operator
If you assign the value of y
to x
:
x = y;
What Happens:
x
now holds the value ofy
, which is10
.y
remains unchanged.
Resulting Values:
x = 10;
y = 10;
Key Points to Note
1. Assignment Operation:
- The
=
operator copies the value from the right-hand side (RHS) to the left-hand side (LHS) variable. - It does not create a link between the variables. Changing one doesn’t affect the other.
2. Variable Values Are Independent:
- After
x = y;
, bothx
andy
contain10
. - Changing
x
later won’t impacty
, and vice versa.
x = 20;
// Now, x = 20 and y = 10
3. Different Data Types:
- The assignment operator works with various data types, not just integers.
Examples:
- String Assignment:
String firstName = "Alice";
String lastName = "Smith";
firstName = lastName; // firstName now holds "Smith"
- Boolean Assignment:
boolean isActive = true;
boolean isVerified = false;
isActive = isVerified; // isActive now holds false
4. Multiple Assignments:
- Chain assignments to assign the same value to multiple variables simultaneously.
int a, b, c;
a = b = c = 50; // All three variables now hold the value 50
5. Reassignment:
- Variables can be reassigned multiple times throughout the program.
int score = 100;
score = 150; // score is now 150
Common Misconceptions
Assignment vs. Equality:
=
is the assignment operator, used to assign values.
==
is the equality operator, used to compare two values for equality.
Example:
int x = 5; // Assignment
boolean isEqual = (x == 5); // Comparison, evaluates to true
Basic Operators in Java
Java allows you to perform standard mathematical operations on variables using basic operators. These operators include +
, -
, *
, /
, and %
, representing addition, subtraction, multiplication, division, and modulus, respectively.
List of Basic Operators
- Addition (
+
) - Subtraction (
-
) - Multiplication (
*
) - Division (
/
) - Modulus (
%
)
Example Scenario
Let’s work with two integer variables:
int x = 5;
int y = 4;
Using these variables, we can perform the following operations:
1. Addition (+
):
int sum = x + y; // sum = 5 + 4 = 9
Result: sum = 9
2. Subtraction (-
):
int difference = x - y; // difference = 5 - 4 = 1
Result: difference = 1
3. Multiplication (*
):
int product = x * y; // product = 5 * 4 = 20
Result: product = 20
4. Division (/
):
int quotient = x / y; // quotient = 5 / 4 = 1
Result: quotient = 1
Note: Since both x
and y
are integers, the division operation results in an integer by discarding the fractional part.
5. Modulus (%
):
int remainder = x % y; // remainder = 5 % 4 = 1
Result: remainder = 1
Understanding Division in Java
Integer Division
When both operands in a division operation are integers, Java performs integer division, which means the result will also be an integer with any fractional part truncated.
int a = 7;
int b = 3;
int result = a / b; // result = 2
Explanation: 7 / 3
equals 2.333...
, but since both a
and b
are integers, the result is truncated to 2
.
Floating-Point Division
If either operand is a floating-point number (float
or double
), Java performs floating-point division, resulting in a decimal value.
double a = 7.0;
int b = 3;
double result = a / b; // result = 2.3333333333333335
Explanation: Here, a
is a double
, so the division retains the fractional part, resulting in approximately 2.3333
.
int a = 7;
double b = 3.0;
double result = a / b; // result = 2.3333333333333335
Explanation: Similarly, if b
is a double
, the division operation yields a floating-point result.
Key Points to Remember
1. Operator Precedence:
Java follows a specific order of operations (precedence) when evaluating expressions. For example, multiplication and division have higher precedence than addition and subtraction.
int result = x + y * 2; // Equivalent to x + (y * 2) = 5 + 8 = 13
2. Type Casting:
To obtain a floating-point result from integer division, you can cast one of the operands to double
or float
.
double accurateQuotient = (double) x / y; // accurateQuotient = 5.0 / 4 = 1.25
3. Modulus Operator (%
):
The modulus operator returns the remainder after division.
int evenCheck = x % 2; // evenCheck = 5 % 2 = 1 (odd number)
4. Chaining Operations:
Combine multiple operations in a single statement, but be mindful of operator precedence.
int combinedResult = (x + y) * 2; // (5 + 4) * 2 = 18
Additional Assignment Operators in Java
Java offers several compound assignment operators like +=
, -=
, *=
, /=
, and %=
. These operators provide a shorthand way to perform an operation and assign the result to a variable simultaneously.
Compound Assignment Operators (+=
, -=
, *=
, /=
, %=
)
Consider a variable x
initialized with a value of 5
:
int x = 5;
If you want to increment x
by 2
, you can write:
x = x + 2;
This statement evaluates the expression on the right side (x + 2
) and assigns the result (7
) back to x
, updating its value from 5
to 7
.
Alternatively, use the +=
operator for the same effect:
x += 2; // Equivalent to x = x + 2;
Similarly, other compound operators work in the same manner:
x -= 2; // Equivalent to x = x - 2;
x *= 2; // Equivalent to x = x * 2;
x /= 2; // Equivalent to x = x / 2;
x %= 2; // Equivalent to x = x % 2;
Increment (++
) and Decrement (--
) Operators
Java also supports the ++
(increment) and --
(decrement) operators, which are used to increase or decrease a variable’s value by 1
without explicitly using the =
operator.
Example of the Increment Operator (++
):
int x = 2;
x++; // Increments x by 1, so x becomes 3
The statement x++;
is functionally equivalent to x = x + 1;
.
Placement of ++
: Prefix vs. Postfix
The ++
operator can be placed either before or after the variable name, and this placement affects the order in which operations are performed.
1. Postfix Increment (index++
):
int index = 5;
System.out.println(index++); // Prints 5
// After this statement, index becomes 6
Explanation: The original value of index
is printed first, and then index
is incremented by 1
.
This sequence is equivalent to:
System.out.println(index);
index = index + 1;
2. Prefix Increment (++index
):
int index = 5;
System.out.println(++index); // Prints 6
// index is already incremented before printing
Explanation: index
is incremented by 1
first, and then the new value is printed.
This sequence is equivalent to:
index = index + 1;
System.out.println(index);
In addition to the ++
operator, Java provides the --
operator to decrease the value of a variable by 1
.
Example of the Decrement Operator (--
):
int y = 10;
y--; // Decrements y by 1, so y becomes 9
Like the ++
operator, --
can be used in both prefix and postfix forms:
1. Postfix Decrement (y--
):
int y = 10;
System.out.println(y--); // Prints 10
// After this statement, y becomes 9
2. Prefix Decrement (--y
):
int y = 10;
System.out.println(--y); // Prints 9
// y is already decremented before printing
Key Points to Remember
1. Shorthand Operations:
- Compound assignment operators (
+=
,-=
,*=
,/=
,%=
) provide a concise way to perform operations and assignments in a single statement.
2. Increment and Decrement Operators:
- The
++
and--
operators are useful for quickly adjusting a variable’s value by1
.
- Placement Matters: Prefix (
++x
) vs. postfix (x++
) affects when the increment or decrement occurs relative to other operations.
3. Operator Precedence:
- Understanding the precedence of operators is crucial, especially when combining multiple operations in a single statement.
Example:
System.out.println(index++); // Postfix ++ has lower precedence than println, prints original value before incrementing
4. Readability and Maintenance:
- While these operators offer shorthand methods, use them judiciously to maintain code readability. Overuse or complex nesting can make code harder to understand.
Type Casting in Java
Sometimes, you’ll need to convert a value from one data type to another, such as converting a double
to an int
. This process is known as type casting. Java handles type casting in two main ways: widening and narrowing conversions.
1. Widening Primitive Conversion
Widening conversion occurs when you convert a smaller data type to a larger one. This type of conversion is automatic and does not require explicit casting because it doesn’t risk data loss.
Example:
short ammo = 30;
double myDoubleAmmo = ammo;
- Explanation:
- A
short
(2 bytes) is automatically converted to adouble
(8 bytes). - This is safe and doesn’t require any special syntax.
- A
Key Points:
- No Data Loss: All possible values of the smaller type can be represented by the larger type.
- Automatic Conversion: Java handles this implicitly without needing explicit casting.
2. Narrowing Primitive Conversion
Narrowing conversion happens when you convert a larger data type to a smaller one. This type of conversion requires explicit casting because it can lead to data loss.
Example:
int a = (int) 25.5;
- Explanation:
- A
double
value25.5
(8 bytes) is explicitly cast to anint
(4 bytes). - The fractional part
.5
is truncated, resulting ina
holding the value25
.
- A
Another Example:
float val1 = (float) 10.5;
- Explanation:
- A
double
value10.5
is explicitly cast to afloat
(4 bytes). - The value
10.5
is preserved accurately in this case, but larger or more precise values may lose precision.
- A
Key Points:
- Potential Data Loss: Since the target type is smaller, some data may be lost during conversion (e.g., decimal points or higher-order bits).
- Explicit Casting Required: Use parentheses to specify the target type.
Incorrect Example (With Corrections):
float val1 = (float) 10.5; // Corrected from (flat) to (float)
- Result:
val1
will hold the value10.5
.
Common Pitfalls
1. Truncation of Decimal Values:
int b = (int) 10.9; // b will be 10, not 11
2. Loss of Precision:
double largeNumber = 123456789.123456789; float smallerNumber = (float) largeNumber; // smallerNumber may lose precision
The float
type cannot accurately represent very large or highly precise double
values.
3. Safe Practices with Type Casting
Prefer Widening When Possible: If your application’s logic allows, opt for widening conversions to maintain data integrity.
Avoid Unnecessary Narrowing: Only perform narrowing conversions when absolutely necessary to prevent unintended data loss.
Use Casting Judiciously: Always be aware of the potential loss of information when casting from a larger to a smaller data type.